CMD Magic
Uncover powerful command-line tricks and tips for Linux, macOS, and shell scripting
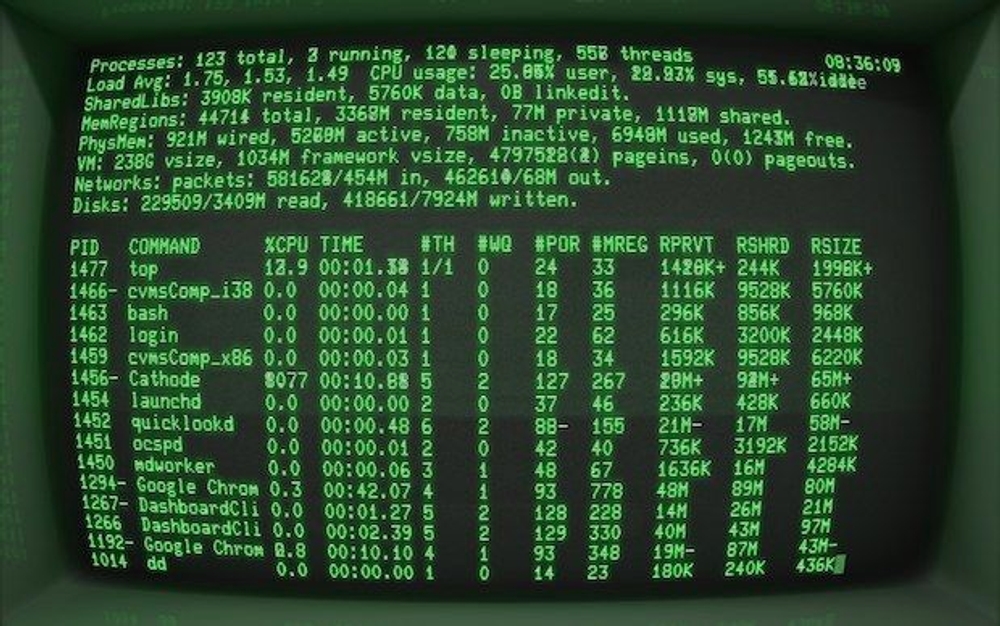
Published
- 4 min read
Last year, I published Vim Magic, a compilation of useful commands for the Vim editor. I’ve been updating it ever since whenever I come across new tips and tricks. It has been a handy reference for me and hopefully for others as well. This post aims to do the same, but for the Linux/Unix command line.
This is not a beginner’s tutorial on how to use the command line. Instead, it’s a reference for those who are already familiar with the terminal and want to learn some new tricks or revisit older ones.
Scripts
#!/bin/bash
Use this as the first line of a bash script to specify the interpreter.
Files
for i in *.png; do convert "$i" "${i%.*}.jpg"; done
Convert all .png files to .jpg.find . -type f -exec grep -El "someWord|someOtherWord" {} \;
Find all files in the current directory (and subdirectories) containingsomeWord
orsomeOtherWord
. Use the escaped semicolon to indicate the end of theexec
command.find . -type f | xargs grep -El "something|appstore"
Similar to the previous command but usesxargs
instead of-exec
.find /path/to/files* -mtime +5 -exec rm {} \;
Delete files older than five days.find . -name '*.zip' -exec unzip {} \;
Unzip all zip files in the current directory.find . -type d | egrep -o '.*src$'
Find directories namedsrc
but exclude their subdirectories.find . 2>/dev/null
Runfind
while suppressingpermission denied
messages.wdiff one.txt two.txt | colordiff
Highlight differences between one.txt and two.txt in color.diff -rq(w) dir1 dir2
Compare two directory structures.SOME_FILE=$(<some-file.txt)
Load the contents of some-file.txt into the variable SOME_FILE.cat oldfile.txt | tr -d '\n' > newfile.txt
Create newfile.txt as a copy of oldfile.txt but with all newlines removed.convert file.{jpg,png}
Equivalent toconvert file.jpg file.png
.
With xargs
, you can avoid loops by passing the output of one command as input to another:
find . -name "*.png" | xargs rm -rf
Recursively delete all .png files.git branch --merged | grep -v \* | xargs git branch -D
Delete all local Git branches that have already been merged.
Users and Groups
cat /etc/passwd | cut -d: -f1
List all users.cat /etc/group | cut -d: -f1
List all groups.chown user file
Change the owner of a file.chgrp group file
Change the group of a file.chown user:group file
Change both the owner and group of a file.
Networking and SSH
lsof -nP | grep 9092
Find the process using port 9092.rsync -avz --remove-source-files -e ssh /local/dir remoteuser@remotehost:/remote/dir
Transfer files via SSH and delete them from the source after copying.ssh user@your-server.com -L 2000:25
Open an SSH session with port tunneling.ssh -Y user@your-server.com
Start an X11 session.sudo route delete default gw 10.0.2.2 eth0
Remove the default gateway oneth0
.sudo route add default gw 192.168.1.254 eth0
Add a default gateway oneth0
.wget -c -t 0 --timeout=60 --waitretry=60 --read-timeout=10 http://example.com/file.mkv
Resume a partially downloaded file (-c
), retry indefinitely (-t 0
), with specific timeouts and retries.wget -r -np -nH -R index.html http://hostname/aaa/bbb/ccc/ddd/
Download all files and subdirectories from theddd
directory, without ascending (-np
), skippingindex.html
files (-R index.html
), and omitting the hostname directory (-nH
).
Random Magic
<Ctrl>+r
Reverse search: search for a previously used command.cal
Display the current month’s calendar.sudo apt-get install build-essential checkinstall
Install essential tools for building packages (for Debian-based systems).openssl base64 -in input.txt -out encoded.b64
Encode input.txt in Base64 and save it as encoded.b64.sudo !!
Re-run the last command withsudo
.$( command )
Capture the output of a command.service --status-all
Display the status of all services.echo "hello world" | sed -e "s/e/o/g" | sed -e "s/lo/a/g" | sed -e "s/world/mundo/g"
Translate “hello world” into “hoa mundo” using multiplesed
substitutions.docker ps | awk '{print $8}'
Display only the names of current Docker containers.
Remote SSH Sessions Without a Password
ssh-keygen -t rsa -b 2048
Generate an RSA key pair.ssh-copy-id youruser@yourserver
Authorize the key on the remote server.ssh youruser@yourserver
Log in without entering a password.